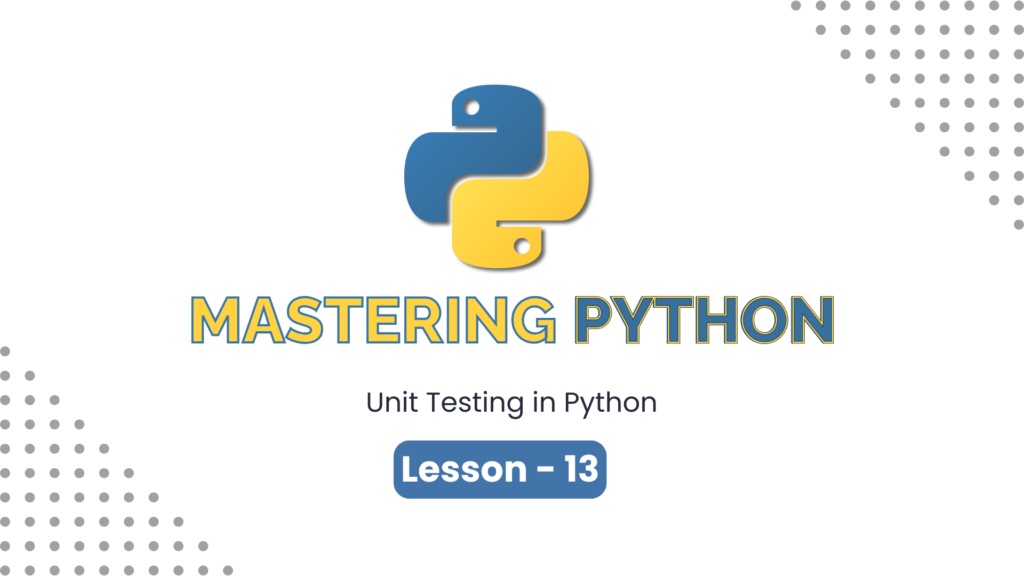
Testing is a crucial part of software development. It ensures that your code is reliable, efficient, and bug-free before deployment. In this lesson, we will explore unit testing in Python using the built-in unittest
module.
1. Why Testing is Important?
What is Unit Testing?
Unit Testing is the process of testing individual functions or components of a program in isolation. It helps developers:
- Identify and fix bugs early.
- Ensure code changes do not break existing functionality.
- Improve software reliability and maintainability.
Types of Testing in Software Development
- Unit Testing – Testing individual functions or components.
- Integration Testing – Testing multiple components working together.
- Functional Testing – Ensuring the application behaves as expected.
- Regression Testing – Checking that new code doesn’t break existing functionality.
Writing Tests Using unittest
Python provides a built-in module called unittest
to create and execute tests.
Installing and Importing unittest
unittest
comes pre-installed with Python, so no additional installation is required.
Basic Test Case Structure
A simple test case consists of:
- A test class that inherits from
unittest.TestCase
. - Test methods that begin with
test_
. - Assertions to check expected outcomes.
Example:
import unittest
# Function to be tested
def add(a, b):
return a + b
# Test Case
class TestMathOperations(unittest.TestCase):
def test_add(self):
self.assertEqual(add(2, 3), 5) # Pass
self.assertEqual(add(-1, 1), 0) # Pass
self.assertEqual(add(0, 0), 0) # Pass
# Run Tests
if __name__ == '__main__':
unittest.main()
2. Running the Test
Save the file as test_math.py
and run it using:
python -m unittest test_math.py
If all tests pass, you will see:
.
----------------------------------------------------------------------
Ran 1 test in 0.001s
OK
3. Assertions and Test Cases
Assertions are used to validate the test results. Some common assertions in unittest
are:
Assertion Method | Description | Example |
---|---|---|
assertEqual(a, b) | Passes if a == b | assertEqual(2+3, 5) |
assertNotEqual(a, b) | Passes if a != b | assertNotEqual(2+2, 5) |
assertTrue(x) | Passes if x is True | assertTrue(5 > 3) |
assertFalse(x) | Passes if x is False | assertFalse(3 > 5) |
assertIn(a, b) | Passes if a is in b | assertIn('hello', 'hello world') |
assertIsNone(x) | Passes if x is None | assertIsNone(None) |
Example:
class TestStringMethods(unittest.TestCase):
def test_upper(self):
self.assertEqual('python'.upper(), 'PYTHON')
def test_isupper(self):
self.assertTrue('PYTHON'.isupper())
self.assertFalse('Python'.isupper())
def test_split(self):
self.assertEqual('hello world'.split(), ['hello', 'world'])
with self.assertRaises(TypeError):
'hello world'.split(2) # Incorrect usage
4. Test Coverage and Best Practices
Measuring Test Coverage
Test coverage measures how much of the code is covered by tests. Use coverage.py to check coverage:
pip install coverage
coverage run -m unittest discover
coverage report -m
Best Practices for Unit Testing
- Write small, independent tests for each function.
- Use meaningful test names (e.g.,
test_login_valid_user
). - Mock external dependencies using
unittest.mock
. - Ensure high test coverage (at least 80%).
- Run tests frequently during development.
Example using mock
:
from unittest.mock import patch
def fetch_data():
return "Real Data"
class TestMockExample(unittest.TestCase):
@patch('__main__.fetch_data', return_value="Mock Data")
def test_fetch_data(self, mock_fetch):
self.assertEqual(fetch_data(), "Mock Data")
Conclusion
Unit testing in Python using unittest
ensures that your code works correctly and prevents future bugs. Mastering unit testing is essential for writing robust, maintainable, and scalable software.
Next Steps: Explore pytest, mocking, and continuous integration (CI) for automated testing!