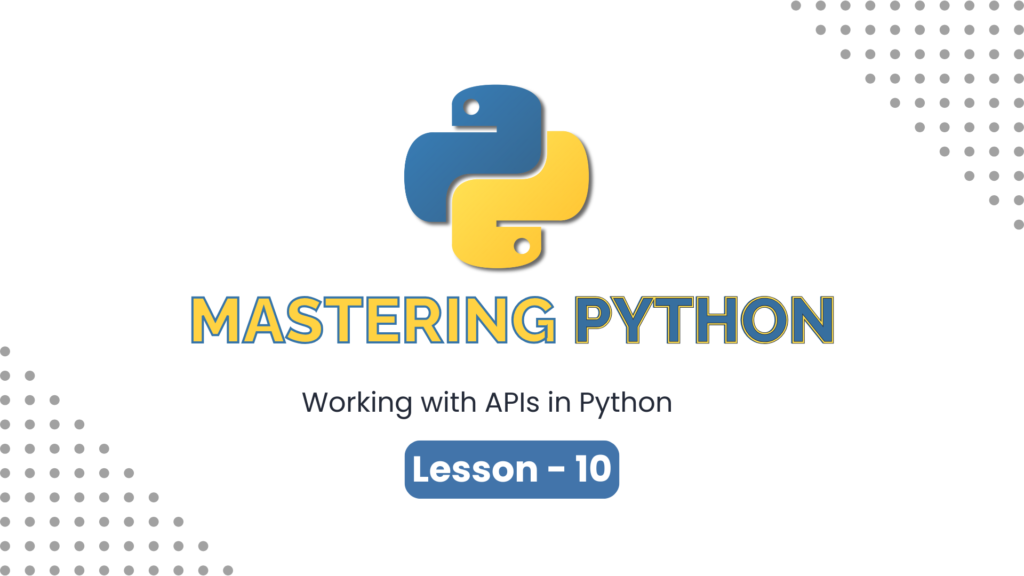
In this lesson, we’ll explore how to interact with APIs in Python, enabling applications to exchange data and functionality over the web.
What is an API?
- API: Application Programming Interface, a set of rules that allows two applications to communicate.
- Types of APIs:
- REST APIs: Use HTTP methods (GET, POST, PUT, DELETE).
- SOAP APIs: Use XML for message exchange (less common in modern use).
- JSON: A common format for exchanging data with APIs.
Setting Up for API Interaction
- Install the
requests
library:
Bash
pip install requests
Use json
library for handling JSON responses.
HTTP Methods in APIs
- GET: Retrieve data.
- POST: Send data to create resources.
- PUT/PATCH: Update resources.
- DELETE: Remove resources.
Making API Requests
1. GET Request
Python
import requests
response = requests.get("https://jsonplaceholder.typicode.com/posts")
if response.status_code == 200:
data = response.json() # Convert JSON to Python dict
print("Data:", data)
else:
print("Failed to fetch data. Status code:", response.status_code)
2. POST Request
Python
import requests
payload = {
"title": "Python API Example",
"body": "This is an example of a POST request.",
"userId": 1
}
response = requests.post("https://jsonplaceholder.typicode.com/posts", json=payload)
if response.status_code == 201: # 201 Created
print("Post created:", response.json())
else:
print("Failed to create post. Status code:", response.status_code)
3. Using Headers
Python
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
response = requests.get("https://api.example.com/data", headers=headers)
Handling Responses
- Status Codes:
200
: OK201
: Created400
: Bad Request401
: Unauthorized404
: Not Found500
: Server Error
- Response Handling:pythonCopy code
Python
if response.status_code == 200:
print("Success:", response.json())
else:
print("Error:", response.status_code)
Error Handling in API Requests
Python
try:
response = requests.get("https://api.example.com/data")
response.raise_for_status() # Raises an HTTPError for bad responses (4xx and 5xx)
data = response.json()
print(data)
except requests.exceptions.HTTPError as err:
print("HTTP Error:", err)
except requests.exceptions.ConnectionError as err:
print("Connection Error:", err)
except requests.exceptions.Timeout as err:
print("Timeout Error:", err)
except requests.exceptions.RequestException as err:
print("Request Error:", err)
Working with Authentication
API Key Authentication:
Python
response = requests.get("https://api.example.com/data?api_key=YOUR_API_KEY")
OAuth2 Authentication:
Python
headers = {"Authorization": "Bearer YOUR_ACCESS_TOKEN"}
response = requests.get("https://api.example.com/secure-data", headers=headers)
Using Third-Party Libraries
http.client
: Low-level HTTP library.Flask
orFastAPI
: For building your own APIs.- GraphQL Clients:
- Use libraries like
gql
.
- Use libraries like
Practical Example: Weather API
Python
import requests
API_KEY = "your_api_key"
BASE_URL = "http://api.openweathermap.org/data/2.5/weather"
city = "London"
params = {"q": city, "appid": API_KEY, "units": "metric"}
response = requests.get(BASE_URL, params=params)
if response.status_code == 200:
data = response.json()
print(f"Weather in {city}: {data['main']['temp']}°C")
else:
print("Failed to fetch weather data.")
Best Practices
- Read the API Documentation: Understand available endpoints, parameters, and limitations.
- Use Environment Variables: Store API keys securely using libraries like
python-dotenv
. - Implement Error Handling: Prepare for network issues, bad responses, and timeouts.
- Rate Limiting: Respect API usage limits to avoid being blocked.
- Logging: Log requests and responses for debugging.
By mastering APIs, you can build powerful applications that interact seamlessly with external services, enabling features like weather updates, payment integrations, and more.