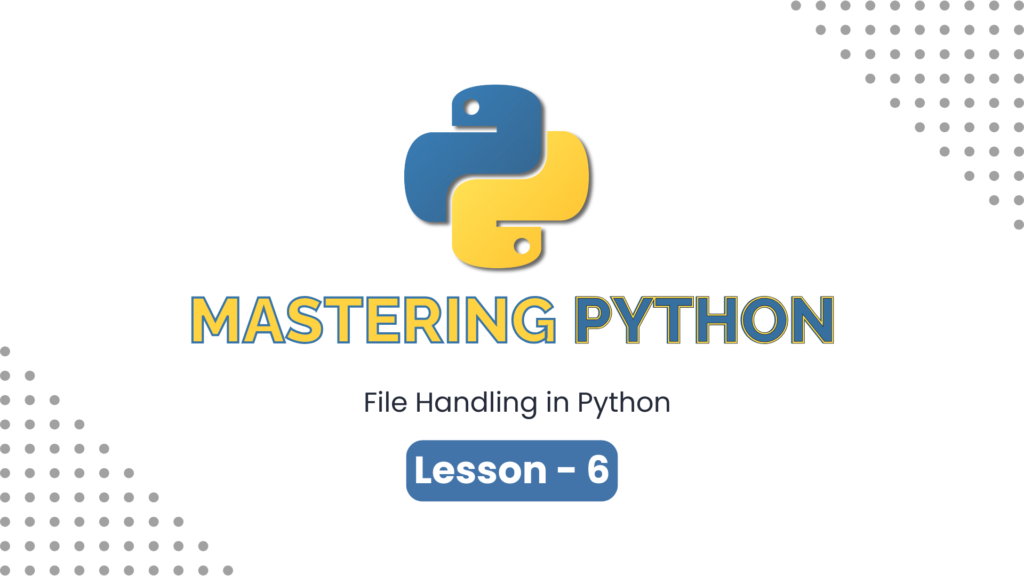
1. Introduction to File Handling
- Understand why file handling is essential in programming.
- Applications include storing data, reading configurations, logging, and data exchange.
2. Modes for File Handling
- r: Read (default) – Opens a file for reading, error if the file does not exist.
- w: Write – Creates a new file or overwrites an existing file.
- a: Append – Adds content to the end of an existing file.
- r+: Read and Write – Allows both reading and writing.
3. Reading Files
- Open a file using the
open()
function. - Examples:
Python
file = open("example.txt", "r")
content = file.read()
print(content)
file.close()
- Reading methods:
read()
: Reads the entire content.readline()
: Reads a single line.readlines()
: Returns a list of all lines.
4. Writing to Files
- Write data using the
write()
method:
Python
file = open("example.txt", "w")
file.write("This is a sample text.")
file.close()
Appending data:
Python
file = open("example.txt", "a")
file.write("\nAdding more text to the file.")
file.close()
5. Using the with
Statement
- Best practice to handle files, ensuring proper closure:
Python
with open("example.txt", "r") as file:
content = file.read()
print(content)
6. Handling Exceptions
- Use
try-except
to manage errors like missing files:
Python
try:
with open("nonexistent.txt", "r") as file:
content = file.read()
except FileNotFoundError:
print("The file does not exist.")
7. Working with File Paths
- Handle file paths using the
os
andpathlib
modules:
Python
import os
print(os.path.abspath("example.txt"))
8. Reading and Writing CSV Files
- Use the
csv
module for structured data