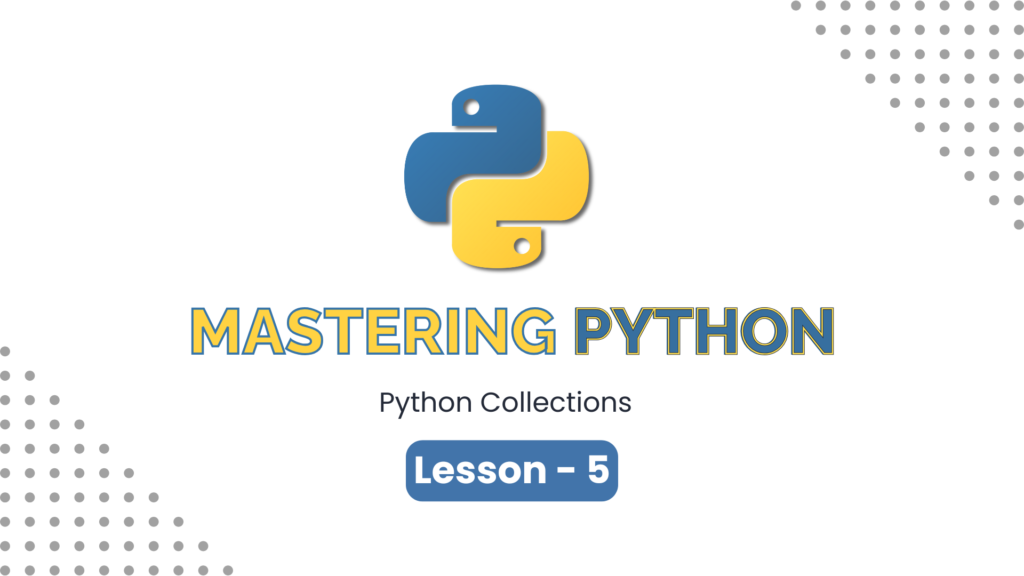
Python collections are data structures that allow you to store and manipulate groups of data effectively. In this lesson, we’ll explore the four primary types of collections in Python: Lists, Tuples, Sets, and Dictionaries.
1. Lists
Lists are ordered, mutable collections that can hold a variety of data types.
Key Features:
- Ordered: Elements maintain their insertion order.
- Mutable: You can change elements after creation.
- Allow duplicates.
Syntax:
my_list = [1, 2, 3, 4, 5]
Common Operations:
# Accessing elements
print(my_list[0]) # Output: 1
# Adding elements
my_list.append(6)
# Removing elements
my_list.remove(3)
# Iterating
for item in my_list:
print(item)
2. Tuples
Tuples are ordered, immutable collections. They are useful for storing fixed sets of data.
Key Features:
- Ordered: Elements maintain their order.
- Immutable: Cannot be changed after creation.
- Allow duplicates.
Syntax:
my_tuple = (1, 2, 3, 4, 5)
Common Operations:
# Accessing elements
print(my_tuple[1]) # Output: 2
# Slicing
print(my_tuple[1:3]) # Output: (2, 3)
3. Sets
Sets are unordered, mutable collections of unique elements.
Key Features:
- Unordered: Elements do not have a defined order.
- Mutable: Elements can be added or removed.
- Do not allow duplicates.
Syntax:
my_set = {1, 2, 3, 4, 5}
Common Operations:
# Adding elements
my_set.add(6)
# Removing elements
my_set.remove(3)
# Set operations
another_set = {4, 5, 6}
print(my_set.union(another_set)) # {1, 2, 3, 4, 5, 6}
print(my_set.intersection(another_set)) # {4, 5}
4. Dictionaries
Dictionaries are collections of key-value pairs.
Key Features:
- Unordered: Elements do not maintain order (in older versions of Python).
- Keys must be unique.
- Mutable: Can be changed after creation.
Syntax:
my_dict = {"name": "Alice", "age": 25, "city": "New York"}
Common Operations:
# Accessing values
print(my_dict["name"]) # Output: Alice
# Adding key-value pairs
my_dict["job"] = "Engineer"
# Removing key-value pairs
del my_dict["age"]
# Iterating through keys and values
for key, value in my_dict.items():
print(f"{key}: {value}")
Comparison of Collections
Feature | List | Tuple | Set | Dictionary |
---|---|---|---|---|
Ordered | Yes | Yes | No | Yes (Python 3.7+) |
Mutable | Yes | No | Yes | Yes |
Duplicates | Allowed | Allowed | Not Allowed | Keys: Not Allowed |
Conclusion
Understanding Python collections is essential for managing data effectively. Each type of collection has its unique strengths and use cases, enabling you to choose the right tool for your programming needs.
In the next lesson, we’ll explore File Handling in Python, learning how to work with files to read, write, and manage data. Stay tuned!