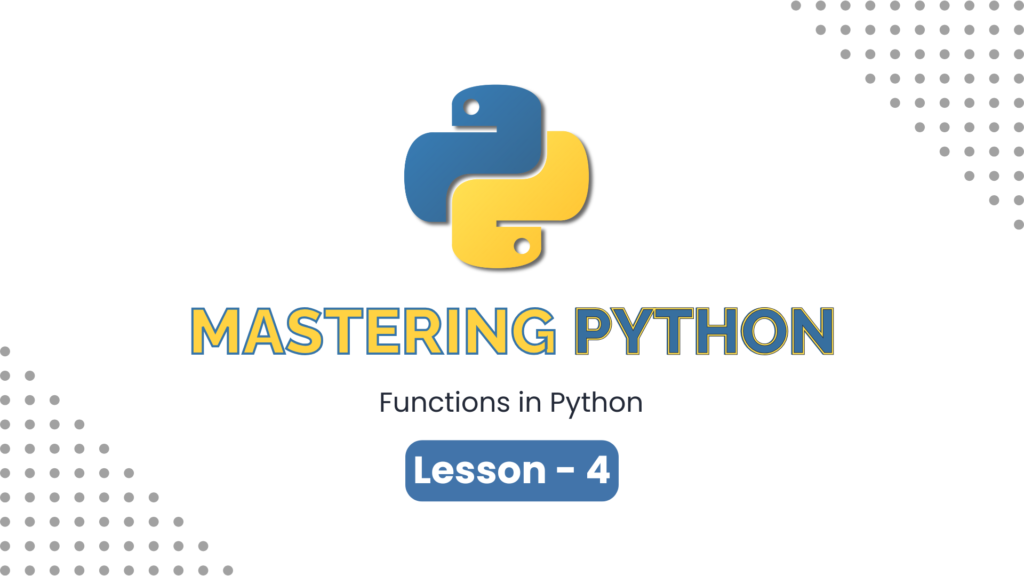
Functions are one of the most important building blocks in Python programming. They allow you to write reusable, modular, and organized code. In this lesson, we’ll cover the basics of defining, calling, and working with functions.
1. What Are Functions?
A function is a block of organized, reusable code that performs a specific task. Functions help to:
- Reduce code duplication.
- Improve code readability.
- Make your program more maintainable.
2. Defining and Calling Functions
You can define a function using the def
keyword and call it by its name.
Syntax:
def function_name(parameters):
# Function body
return value # Optional
Example:
def greet(name):
print(f"Hello, {name}!")
greet("Alice") # Output: Hello, Alice!
3. Parameters and Arguments
Functions can take parameters to customize their behavior.
Example:
def add_numbers(a, b):
return a + b
result = add_numbers(5, 3)
print(result) # Output: 8
Positional Arguments: Passed in the order they are defined.
Keyword Arguments: Passed by explicitly specifying parameter names.
add_numbers(a=5, b=3)
4. Default Parameters
You can define default values for parameters, making them optional.
Example:
def greet(name="Guest"):
print(f"Hello, {name}!")
greet() # Output: Hello, Guest!
greet("Alice") # Output: Hello, Alice!
5. Return Statement
The return
statement is used to send a result back to the caller.
Example:
def square(number):
return number * number
result = square(4)
print(result) # Output: 16
6. Scope of Variables
Variables inside a function are local to that function unless declared global.
Example:
def test_scope():
x = 10 # Local variable
print(x)
test_scope()
# print(x) # Error: x is not defined outside the function
7. Lambda Functions
Lambda functions are anonymous functions defined using the lambda
keyword. They are useful for short, simple operations.
Syntax:
lambda arguments: expression
Example:
square = lambda x: x * x
print(square(5)) # Output: 25
8. Function Documentation (Docstrings)
Use docstrings to describe what a function does.
Example:
def greet(name):
"""
This function greets the user by their name.
"""
print(f"Hello, {name}!")
help(greet) # Displays the docstring
9. Built-in Functions
Python comes with many built-in functions like len()
, max()
, min()
, and sum()
.
Example:
numbers = [1, 2, 3, 4, 5]
print(len(numbers)) # Output: 5
print(sum(numbers)) # Output: 15
Conclusion
In this lesson, we explored how to define, call, and work with functions in Python. Functions enable code reusability and help you write cleaner and more efficient programs. Up next, we’ll learn about Python Collections (Lists, Tuples, Sets, and Dictionaries) to organize and manipulate data effectively!